In Flutter, you can add multiple floating action buttons (FABs) to a single screen by using the Stack widget to position them on top of each other.
Here’s an example code snippet that demonstrates how to add multiple FABs in a Flutter app:
import 'package:flutter/material.dart';
void main() {
runApp( MyFABsScreen());
}
class MyFABsScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('flutterfever FABs'),
),
body: Center(
child: Text('flutterfever Screen'),
),
floatingActionButton: Stack(
children: [
Positioned(
bottom: 80.0,
right: 20.0,
child: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
),
Positioned(
bottom: 20.0,
right: 20.0,
child: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.edit),
),
),
Positioned(
bottom: 100.0,
right: 20.0,
child: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.person),
),
),
],
),
),
);
}
}
output:
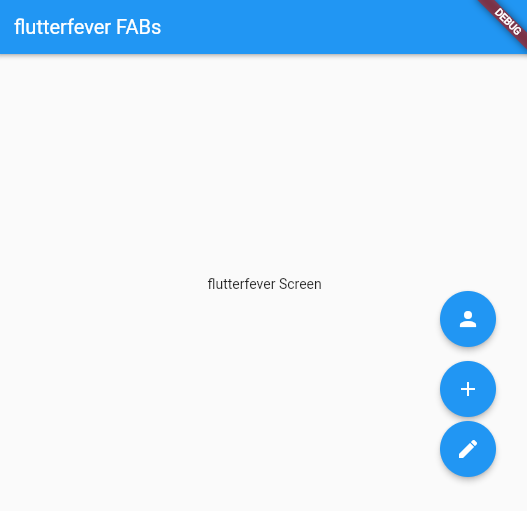
In the code above, we added a Stack widget as the floatingActionButton of the Scaffold widget. Inside the Stack, we added two Positioned widgets, each representing a FAB.
We used the bottom and right properties of the Positioned widget to position the FABs relative to the bottom right corner of the screen. You can adjust these properties to position the FABs anywhere on the screen.
Inside each FloatingActionButton, we defined the onPressed and child properties. The onPressed property is a callback function that will be called when the user taps on the FAB, while the child property is used to define the icon that will be displayed on the FAB.
By using the Stack widget and Positioned widget, we can add multiple FABs to a single screen and position them anywhere on the screen.