Your application’s user experience is a crucial feature that distinguishes your application from other applications that provide the same services, and may help convince users to prefer your app over those others.
One of the approaches to improving your user experience is through custom, advanced animations, and this article is here to guide you through creating them. Here, you’ll learn about some of the advanced animations available in Flutter and how you can implement them in your applications, such as:
- Building simple animations with the
Tween
class - Building complex, staggered animations
- Configuring one controller for animation order management
- Building routing animations in Flutter
- Building shake or bounce animations
- Building animations with Flutter Hooks
- Building themed animation transitions
As you will see in this article, there are easier ways to create Flutter animations. However, the reason we went with the Ticker + Tween + Controller method in our previous article is that it forms the base of all Flutter animations. Even the most complex animations are just variants and combinations of the same type of animation method.
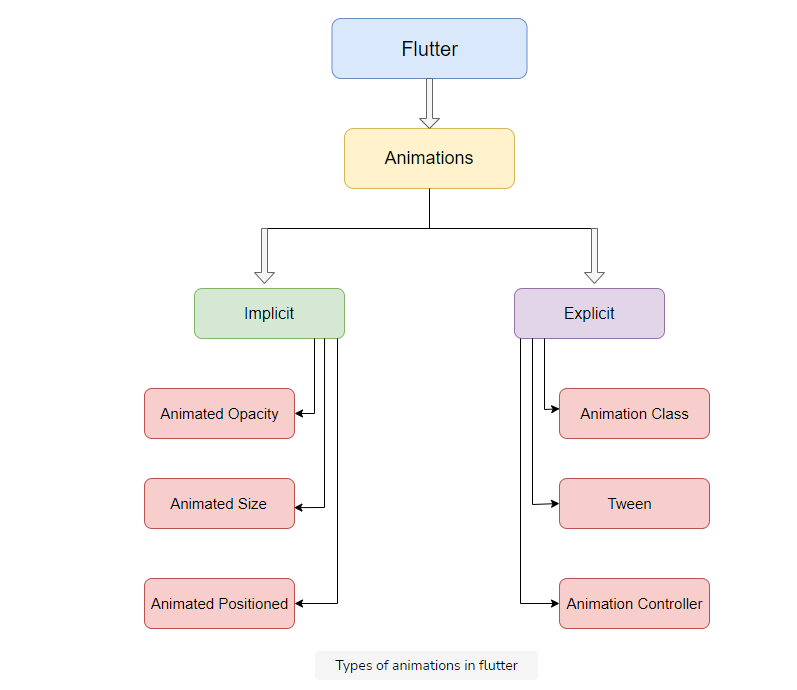
Implicitly Animated Widgets
When we call something ‘implicit’, we mean a quality is inherent in something. In the case of animations, i.e. for implicitly animated widgets, we can effectively say that the code is implicit, meaning it is in-built and does not have to be manually written.
Implicitly Animated Widgets are widgets that automatically animate changes in themselves.
Explicit Widget
These widgets provide a more granular control on the animated widget. They have the properties to control the repetition and movement of the widget. These widgets require an AnimationController for the granular control they provide. This controller can be defined in the initState and the dispose of states for better usage. The explicit widget can be categorized as
- XYZTransition: Here XYZ is a specific widget available as Transition. These are built-in transition which provides more control over the implicit animation. They can be thought of as an extension of the AnimatedXYZ widget. Some explicit XYZTransition available are:
- SizeTransition
- FadeTransition
- AlignTransition
- RotationTransition
- PositionedTransition
- DecoratedBoxTransition
- AnimatedBuilder/ AnimatedWidget: When there are no widgets available from the predefined XYZTransition which already define a variety of Animation then we can use the AnimatedBuilder/ AnimatedWidget. They apply to a custom widget that we want to explicitly animate. If we can define the animation in the same widget then we can use the AnimatedBuilder otherwise if we define a while new widget we can extend the defined widget with the Animated Widget.
Explicit animations
Explicit animations in Flutter require developers to manually specify the animation’s start and end states, as well as the interpolation curve.
This level of control provides greater flexibility and precision, allowing developers to create custom animations according to their own preferences. Flutter offers animation controller classes, such as
AnimationController
Tween
Advantages of explicit animations
- Customization: Explicit animations enable developers to customize the animation’s behavior and appearance in detail, resulting in a more personalized and polished user experience.
- Complex animations: When dealing with difficult animations that involve multiple properties and intermediate steps, explicit animations offer a more suitable approach.
Use cases of explicit animations
- Interactive animations: Explicit animations are ideal for building interactive animations that respond to user input or gesture-based interactions.
- Complex transitions: When you need to create sophisticated animations that span across multiple screens or involve dynamic content, explicit animations provide the level of control required.
Comparison: implicit vs. explicit animations
The differences between these two types of animations can be crucial to understand in order to use them in your application.
Ease of implementation
Implicit animations are beginner-friendly due to their simplicity, as they require minimal setup and configuration. On the other hand, explicit animations demand more coding effort and expertise, making them better suited for experienced developers.
Level of control
Implicit animations abstract much of the animation process, providing limited control over the animation’s behavior. In contrast, explicit animations offer complete control over the animation’s timing, curves, and intermediate states.
Animation performance
Implicit animations are generally more efficient in performance, as Flutter optimizes the rendering process. If not implemented carefully, explicit animations may lead to performance issues, especially with complex animations.
Animation types
Implicit animations are well-suited for simple property-based transitions, like opacity and size changes. Explicit animations, however, can handle both property-based animations and complex transitions involving multiple properties simultaneously.
Reusability
Implicit animations are generally easier to reuse across different parts of an application since their logic is encapsulated within the widget itself. Once an implicit animation widget is defined, it can be placed anywhere in the widget tree without much modification. On the other hand, while explicit animations can be reused, they often require more customization based on the context in which they are used. The custom nature of explicit animations might make them less reusable in different parts of the app.
Learning curve
Due to their simplicity, implicit animations have a lower learning curve, making them accessible to developers who are relatively new to Flutter or animation concepts. However, learning to work with explicit animations may take more time and effort, particularly for those without prior experience in managing animations with the AnimationController
, Tween
, and curves
.