To add dynamic rows to dynamic columns in Flutter, you can use nested ListView.builder
widgets.
Here’s a basic example demonstrating how you can achieve this:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Dynamic Rows and Columns Example'),
),
body: DynamicTable(),
),
);
}
}
class DynamicTable extends StatefulWidget {
@override
_DynamicTableState createState() => _DynamicTableState();
}
class _DynamicTableState extends State<DynamicTable> {
List<List<String>> _data = [
['Row 1, Column 1', 'Row 1, Column 2', 'Row 1, Column 3'],
['Row 2, Column 1', 'Row 2, Column 2', 'Row 2, Column 3'],
];
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: _data.length,
itemBuilder: (context, index) {
return Row(
children: _data[index]
.map((cellData) => Expanded(
child: Container(
decoration: BoxDecoration(
border: Border.all(),
),
padding: EdgeInsets.all(8.0),
child: Text(cellData),
),
))
.toList(),
);
},
);
}
}
Output:
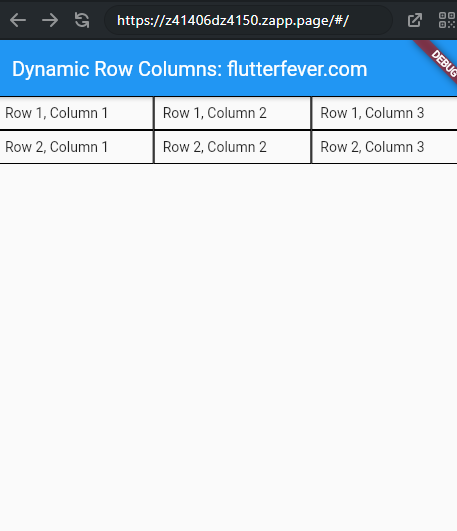
In this example:
- We have a
DynamicTable
widget that is aStatefulWidget
. - The
_data
list contains lists of strings, each representing a row of data. - In the
build
method of_DynamicTableState
, we use aListView.builder
to build dynamic rows. - Inside the
ListView.builder
, we use aRow
widget to represent each row. - For each row, we map over the list of strings in
_data[index]
to build dynamic columns usingExpanded
widgets. - Each column contains a
Container
with border decoration and padding, containing the text data.
This way, you can dynamically generate rows and columns based on your data. You can modify _data
to hold your dynamic data, and the UI will adjust accordingly.